Basics
When you write a host application that consumes the HotDocs Cloud Services web service, you must understand some basic characteristics of the methods you will call. Each HDCS method has a set of common parameters, as well as method-specific parameters. The common parameters, such as the subscriberID, hmac, and packageID, are used to verify that the call is being made by a valid HDCS subscriber, and to locate the template to use with the request in the HotDocs Cloud Services template cache. Before you can call any methods of the HotDocs Cloud Services web service, you must understand the basics of how template packages are cached and how to securely make calls to the HDCS service by calculating a valid Hash-based Message Authentication Code (HMAC) for each call. Sending a template package at the wrong time, not sending it at the right time, or sending an incorrect HMAC value will all prevent you from receiving the desired response from your request. The following sections explain some of fundamental parts of HotDocs Cloud Services:
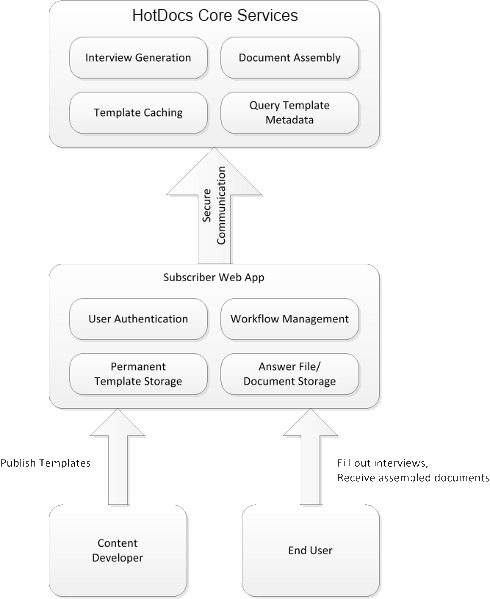
Referencing the HotDocs Cloud Services Web Service
You can either interact with the web service directly, or if you are writing your host application in .NET, you can use the HDCS client library, which simplifies much of the interaction between your host application and HDCS.
To add a service reference to the HDCS web service in Visual Studio
- In Solution Explorer, right-click the name of the project to which you want to add the service, and then select Add Service Reference from the shortcut menu. The Add Service Reference dialog box appears.
- In the Address box, enter https://core.hotdocs.ws/Core.svc and then click Go. The list of services available at that URL is shown in the Services list.
- In the Services list, select the Core > ICore service contract.
- In the Namespace box, enter the namespace that you want to use for the reference, such as HDCoreService.
- Click OK. A reference to the service is added to your project.
To add a reference to the HDCS client library in Visual Studio
- In Solution Explorer, right-click the name of the project to which you want to add the service, and then select Add Reference from the shortcut menu. The Add Reference dialog box appears.
- At the Browse tab, navigate to the location where you have saved HotDocs.Core.Client.dll, and then click OK. A reference to the HDCS client library is added to your project.
Template Package Caching
To reduce bandwidth requirements and improve performance, HotDocs Cloud Services maintains a cache of recently-used template packages. Your host application is responsible for the permanent storage of template packages, and should be prepared to send the package to HotDocs Cloud Services any time it calls one of the web methods. However, it should only send the package if HotDocs Cloud Services throws an exception indicating that the specified template package is not already found in its cache; if you send a package that is already in the cache, HotDocs Cloud Services will throw an exception because it will not know what to do with the duplicate package.
If you update the files in a template package, you must use a new packageID since there is no way to replace one package with a new version of the same package in the template cache. (Once a designated time period has elapsed in which the old package has not been used, HotDocs Cloud Services will remove it from the template cache.)
The following steps illustrate how to make a request to one of the methods of the HotDocs Cloud Services web service:
- In a Try block, call the web method without sending the package (use a null value for the
templatePackage parameter). - If a "HotDocs.Cloud.Storage.PackageNotFoundException" exception occurs, repeat the request, but send the package this time.
If you use the HDCS client library, it has the logic for determining when to send the package or not built-in, which eliminates the need for you to include this logic in your own host application.
Calculating the Hash-based Authentication Code (HMAC)
One of the security measures put into place to guard against unauthorized or fraudulent access to the web service is the HMAC parameter in each method. This is a way to sign the request when it is sent and then let HotDocs Cloud Services verify that signature to be sure that what is received matches what was sent.
To calculate the HMAC
- Get a string representation for each of the parameters that is to be included in the HMAC. Only certain parameters are included in the HMAC calculation, and the order of the parameters is different than the order in which they are found in the web methods. Here is the list of parameters for each method to include in the HMAC calculation:
- GetInterview: timestamp, subscriberID, packageID, templateName, *isPackageIncluded, billingRef, format, tempImageUrl, settings
-
AssembleDocument: timestamp, subscriberID, packageID, templateName, *isPackageIncluded, billingRef, format, settings -
GetComponentInfo: timestamp, subscriberID, packageID, templateName, *isPackageIncluded, billingRef, includeDialogs -
GetAnswers:
* The isPackageIncluded parameter is a boolean value that indicates whether or not the template package is being sent with the request. The other parameters are described in more detail in the API reference sections of this help file. -
Concatenate each parameter into a single string, separated by a line break (\n). -
Use a signing key to compute a hash for the combined string. -
Base-64 encode the hash.
Receiving Template Packages Uploaded from HotDocs Developer
HotDocs Developer contains a plug-in that lets template authors create template packages and upload them to your host application. A template package is a collection of all the files necessary to display an interview or assemble a document for a template. The plug-in automatically scans templates for dependencies that must also be included in the package, such as shared component files, inserted templates, or dialog element images.
Because each template package is "self-sufficient", shared files may exist in multiple template packages. For example, if a common inserted template is updated, you would need to create new template packages for each template that uses that inserted template.
When you publish a template package using HotDocs Developer, you specify the URL of the host application to which you want to publish the template. The host application must be able to accept the template packages. To see how to handle an upload, refer to Upload.aspx in Sample Portal.